Design Patterns and how to understand these Patterns.
25 Apr 2023
How to understand Design Patterns as easy as possible
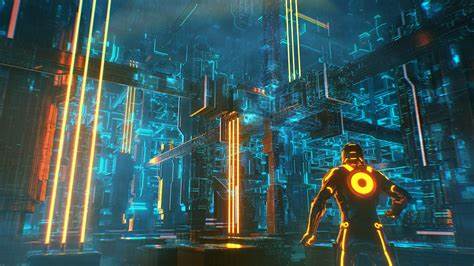
What are Design Patterns?
Design is crucial everywhere whether it’s in art, architecture, engineering, science, and even cooking. In software engineering, design patterns are ways for us to find a solution to a problem and design it in our code in the most efficient way possible. Design patterns require not just logic but creativity as well and it takes many years of practice to be good at it. In fact, many experienced engineers gave their input on how to make great design patterns, and their methods are used by many engineers today.
Types of Design Patterns
There are three types of design patterns:
- Creational: Which are patterns designed for class instantiation.
- Structural: This is used to increase the functionality of the classes without changing the composition.
- Behavioral: Patterns designed depending on the class and how it communicates with others.
Examples of Design Patterns
Here are a few examples of design patterns in software engineering:
- Singleton: A type of object that can only be instantiated once. When we are designing a class we would make the constructor private to prevent new objects from being created thus only allowing us to create one object.
- Prototype: Allows us to implement inherent from objects rather than class. This makes the code less confusing and inheriting more straightforward. By using Object.create and passing the prototype to the object you can create a copy.
- Builder: We can create objects step by step instead of using a constructor. This can be useful when we want to have more control. So instead of using a constructor, we can use method changing.
- Factory: We can use a function or method to instantiate an object rather than the new keyword.
- Facade: API to hide other low-level details in our codebase. So by creating a facade we contain the low-level systems as dependencies and simplify their operation.